FAQs, Troubleshooting & Best Practices
Best Practices
To provide you with the best possible experience, IFX will continue to provide guidelines for an optimal, performant integration.
Integration Size Considerations
It is recommended to create separate integrations based on their type and purpose. For instance, have one integration containing all handlers for Arc Subscription transactional emails, and another to handle story auto-tagging.
Synchronous and asynchronous handlers should not be mixed. This will affect user experience.
Example | Impact | |||
---|---|---|---|---|
|
| |||
|
|
Rate Limits on Arc XP services
Calls to Arc XP services from IFX have rate limits which can impact performance and eventually lead to errors. As you approach the rate limits, you may notice requests to your integration are slowed down.
When you hit rate limits you will receive an http status 429, with the body below:
<html> <head><title>429 Too Many Requests</title></head> <body> <center><h1>429 Too Many Requests</h1></center> <hr><center>nginx</center> </body></html>
This will throw an exception in the ArcHttpClient as the response will not be JSON.
Integrations
How do I know which version is running on which environment?
Use the List Bundles endpoint to see which bundle is set to live:
GET https://api.{myorg}.arcpublishing.com/ifx/api/v1/admin/bundles/{integrationName}/
An event is not triggering in my integration logs
Is the event enabled for your application? For example, Subscriptions’ verify_email
can be disabled in the UI https://{myorg}.arcpublishing.com/subscriptions/settings/identity/sign-in?site={mysite}
My integration is infinitely looping. How can I stop it?
Unsubscribe to the event that is causing the loop by setting enabled to false:
PUT /ifx/api/v1/admin/events/subscriptions
{ "eventName": "story:update", "enabled": false, "integrationName": "myIntegration"}
Can I receive Content Events locally?
Yes. This is explained on Locally Simulate HTTP Requests .
Why am I not seeing any updates within my integration or logs?
Stop your integration using CTRL + C, then npm run localTestingServer
I’m having issues with my deployment.
Check out these articles for more information:
If you are still having an issue, submit an ACS ticket for more help.
Do I need a single event handler for every event I want to receive ?
No. A single handler can receive multiple events.
In Node
{ "storyUpdateHandler": ["story:create", "story:update"], "storyDeleteHandler": ["story:delete"]}
eventsHandlers.js
includes the handler files defined in eventsRouter.json
const storyUpdateHandler = require('./eventsHandlers/storyUpdateHandler');const storyDeleteHandler = require('./eventsHandlers/storyDeleteHandler');
module.exports = { storyUpdateHandler, storyDeleteHandler,}
”SyntaxError: Cannot use import statement outside a module”
When I run npm run build
I am getting “SyntaxError: Cannot use import statement outside a module”
If this is calling out something inside of /node_modules/
, run rm -rf node_modules && npm install
Environments
What environments are available to my integrations?
Sandbox and Production.
Performance and Error Handling
Can I get alerts when my integration fails?
Yes! When you create an integration, part of the payload is email
. In order for alarms to be triggered, you must handle errors in your code properly. Console logs in your code can give details about where the code is failing and will be helpful in debugging. You could also build a helper function that sends custom emails or messages to a Slack channel.
Here is example code where I forced an error and did get an email:
const myHandler = async (event) => { console.log("in myHandler function") try { somethingfun(); } catch (e) { console.error(e); }}async function somethingfun() { throw new Error("Errors are fun!")}
module.exports = myHandler
Error message in local:
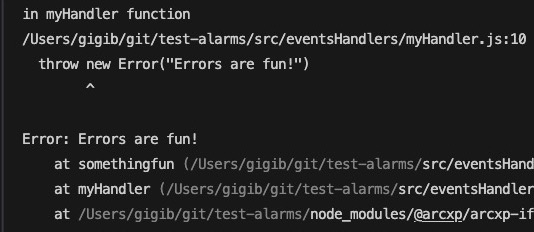
After I deployed and promoted this bundle to sandbox and triggered the event, I received this email:
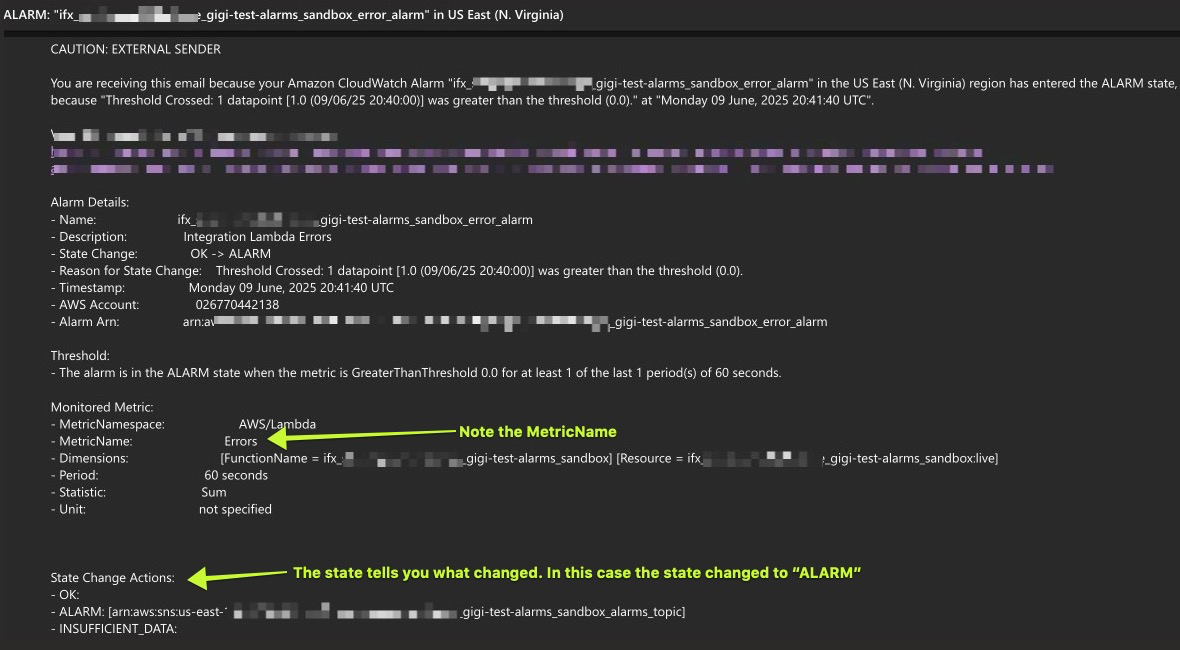
Why am I getting Cloudwatch Alarm Emails?
IFX requires an email address as part of the new integration Create API. The email address provided is used to assign to a set of default Cloudwatch Alarms. We create these alarms for you in an effort to notify in case of an issue.
Most of the emails are informational - if your integration starts taking longer than it usually does, or sees a spike in error results on it’s invocation, we will send the corresponding email address an alarm alert.
How you choose to deal with this info is up to you. If you decide it is not helpful you can unsubscribe from that alarm using the link included in the email.
Is my integration’s performance dependent on other traffic in the network?
No. All processes, even build & deploy jobs, are unaffected by other integrations’ processes.
Logging
Follow integration runtime logs for adding logs to your integration.
Timeouts
My integration keeps timing out. What can I do?
There can be several factors that affect this. Usually it may be due to an external service’s longer response time.
More info on troubleshooting timeouts
It is recommended that you put synchronous and asynchronous event handlers into separate integrations. Why?
- Asynchronous events are the “fire and forget” kind, and have little to no effect on the user experience. Since it is sitting there waiting on a response, sync events do effect user experience. Therefore you do not want async events clogging up your pipeline, delaying sync event response times.
- You can deploy individual integrations separately, leaving other integrations unaffected.
- Since async and sync events behave differently, having the debugging/logging/metrics separate makes it easier.
My integration needs to wait for something to happen
If you are seeing portions of your code running but not the rest, you are likely missing async
declarations.
Make sure you are using async declarations and promises where necessary to ensure your function will wait for a process that might take long. Also, implement try/catch for subsequent API calls.
const timeoutTestHandler = async (event) => { console.log('starting timeout handler'); const result = await myLongFunction(); console.log(`result: ${result}`);};
function myLongFunction() { return new Promise((resolve) => { setTimeout(() => { resolve('resolved'); }, 30000); // wait 30s });}
module.exports = timeoutTestHandler;
We can see in these logs that result:resolved
is logged 30 seconds after starting timeout handler
Understanding Async Event Handling & Retries
Event Retries
If your asynchronous event handler experiences an error and does not successfully process an event, the system will automatically retry delivering the event up to two additional times. It’s crucial to design your handlers to gracefully manage potential failures.
Note that if your code utilizes a top level try/catch statement to catch any errors, this automatic retry functionality will not occur.
More-Than-Once Delivery
In certain error scenarios, an asynchronous event might be delivered to your handler more than once. For detailed information about when this can occur, refer to the AWS documentation on Asynchronous Invocation: Asynchronous invocation - AWS Lambda. Designing your event handlers with idempotency is essential.
Idempotency Best Practices
Idempotent operations produce the same result whether executed once or multiple times. To ensure robust asynchronous event handling, adhere to idempotency principles. Examples can be found on Make a Lambda function idempotent.
Common Questions
Can we use IFX to write events to our own Kinesis streams?
This is not supported.
Can we modify the ANS payload using the Draft API and store any environment variables needed for IFX?
You can adjust the ANS in your integration code using the Draft API. For storing environment variables create a file in the root of your project called .env
inside of that file define variables as needed.
Be sure you do not create an infinite loop!
Is it guaranteed that events will arrive in order?
While strict ordering can’t be guaranteed, it’s unlikely for events to arrive out of order in normal conditions. The image below demonstrates quickly updating a story where events arrived in the correct order.
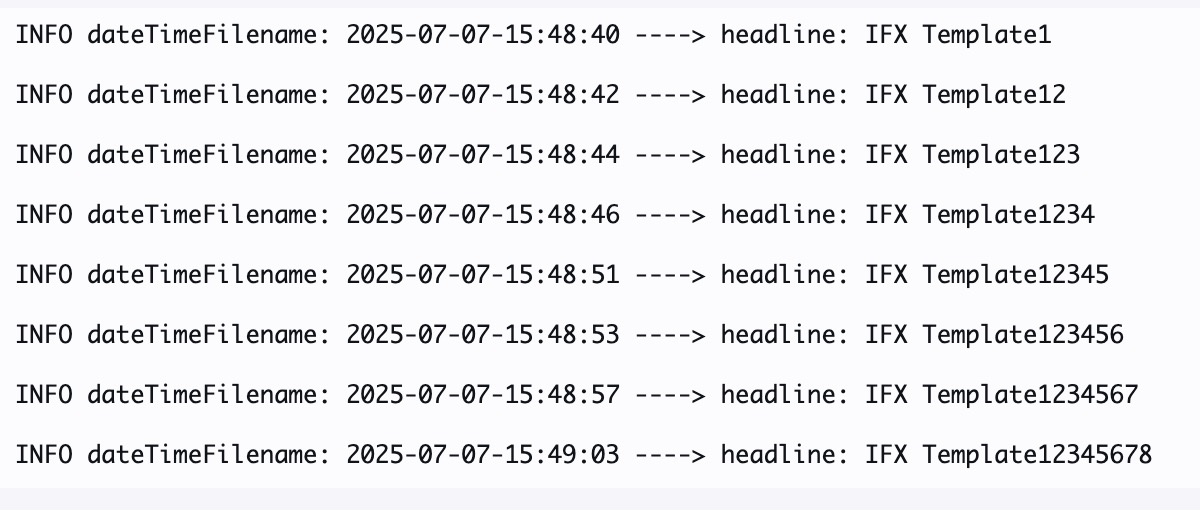
Debugging: Have you checked…
- If an integration is not triggering, does the name of the handler annotation match the integration key?
- If an integration is not triggering, is the integration enabled when you use the List Integrations endpoint to see the status of all your integrations?
- If you are receiving a HTTP Status Code 401, is your Developer PAT still valid?
- If you are receiving an error, can you reproduce reproduce it locally using a build of the same Git tag?
- Have you checked your integration runtime logs?
If an error can’t be reproduced locally or if further research into a problem is needed, you may submit an ACS request.
Help us improve our documentation! Let us know if you do not find what you are looking for by posting suggestions to Our Ideas Portal.