Pushing Arc XP content to Apple News
Background
You can propagate published content from Arc XP into Apple News by using Arc XP’s Integration Framework (IFX). These step-by-step instructions will guide you through using the Apple News API as well as the interactions with Arc XP that you need to get started publishing content to Apple News.
When you adopt this pattern, you have the capability of updating your Apple News articles automatically when performing publishing actions within Arc XP, making Arc XP the single source of truth for all of your articles.
In order to implement this solution, it is crucial that you have at least a basic understanding of how IFX works. If you have not done so already, we recommend you read the documentation.
How the Apple News integration Works
If you’re familiar with IFX, you know that an IFX integration gets invoked when specific events occur. In the case of this integration, the following actions execute the IFX integration:
- Publishing a story
- Updating the publish of a story
- Unpublishing a story
- Republishing a story (publishing a story after an unpublish)
- Deleting a story
Each one of these actions emits an IFX event, which your integration can listen to. Depending on the event, the integration runs a specific handler that contains the necessary logic to perform the corresponding operation in Apple News.
The following table provides several handlers, along with the events each is mapped to:
HANDLERS | EVENT THE HANDLER IS MAPPED TO |
---|---|
storyPublishHandler.js | story:first-publish |
storyRepublishHandler.js | story:update story:republish |
storyUnublishHandler.js | story:unpublish |
storyDeleteHandler.js | story:delete |
You can find this association in the src/eventsRouter.json
file of the integration.
In the following diagrams, you can see the workflow for each one of the handlers.
First Publish Diagram:
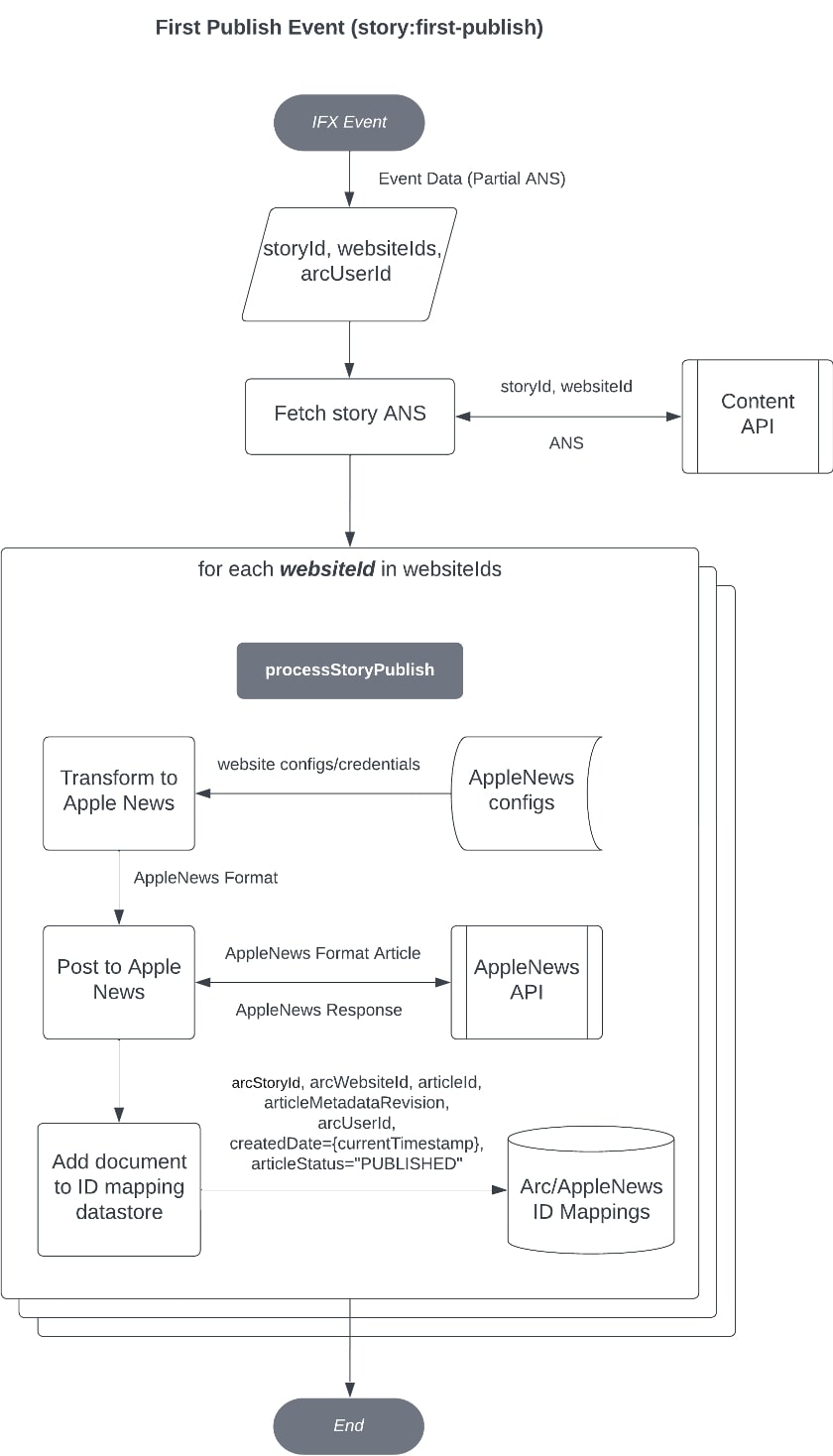
Republish Diagram:
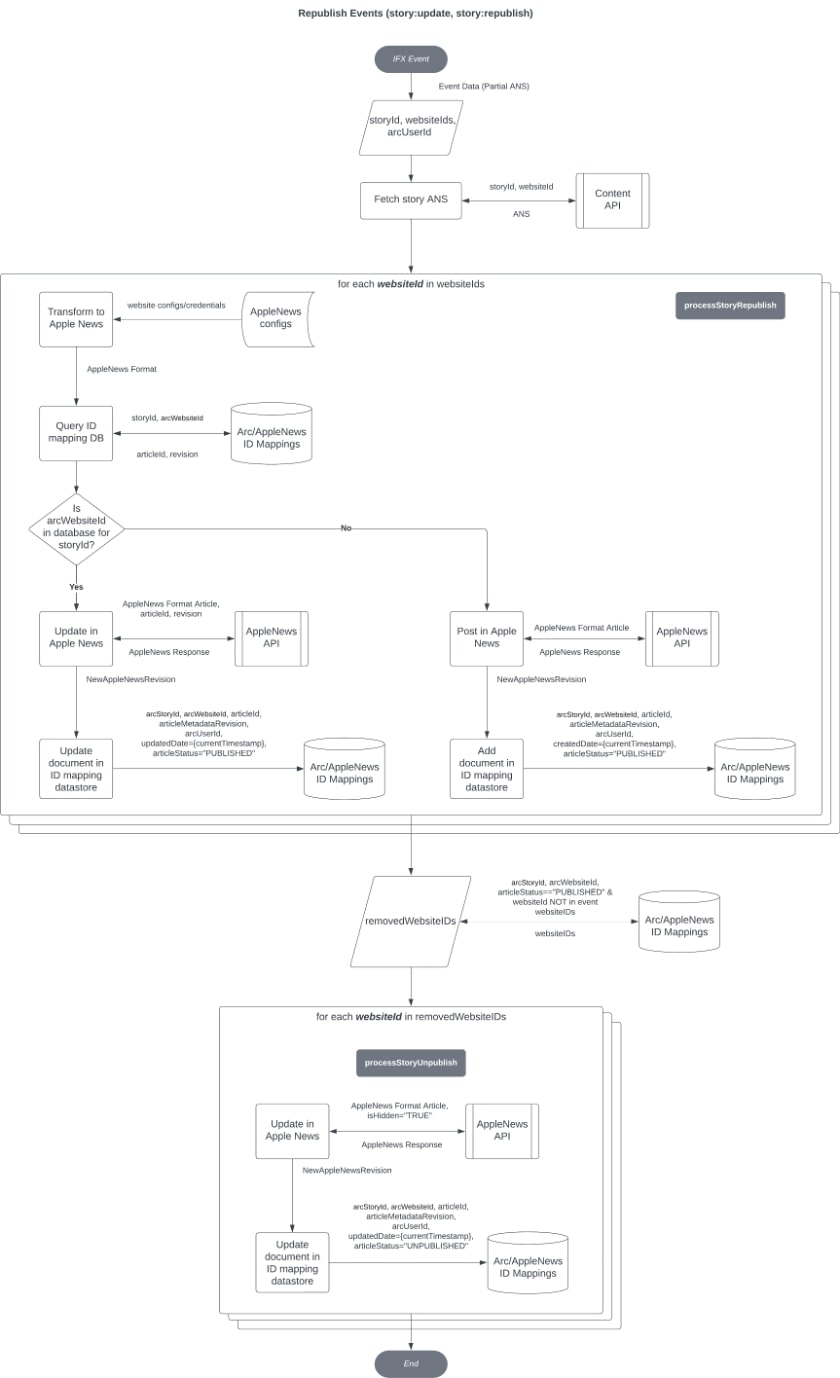
Unpublish Diagram:
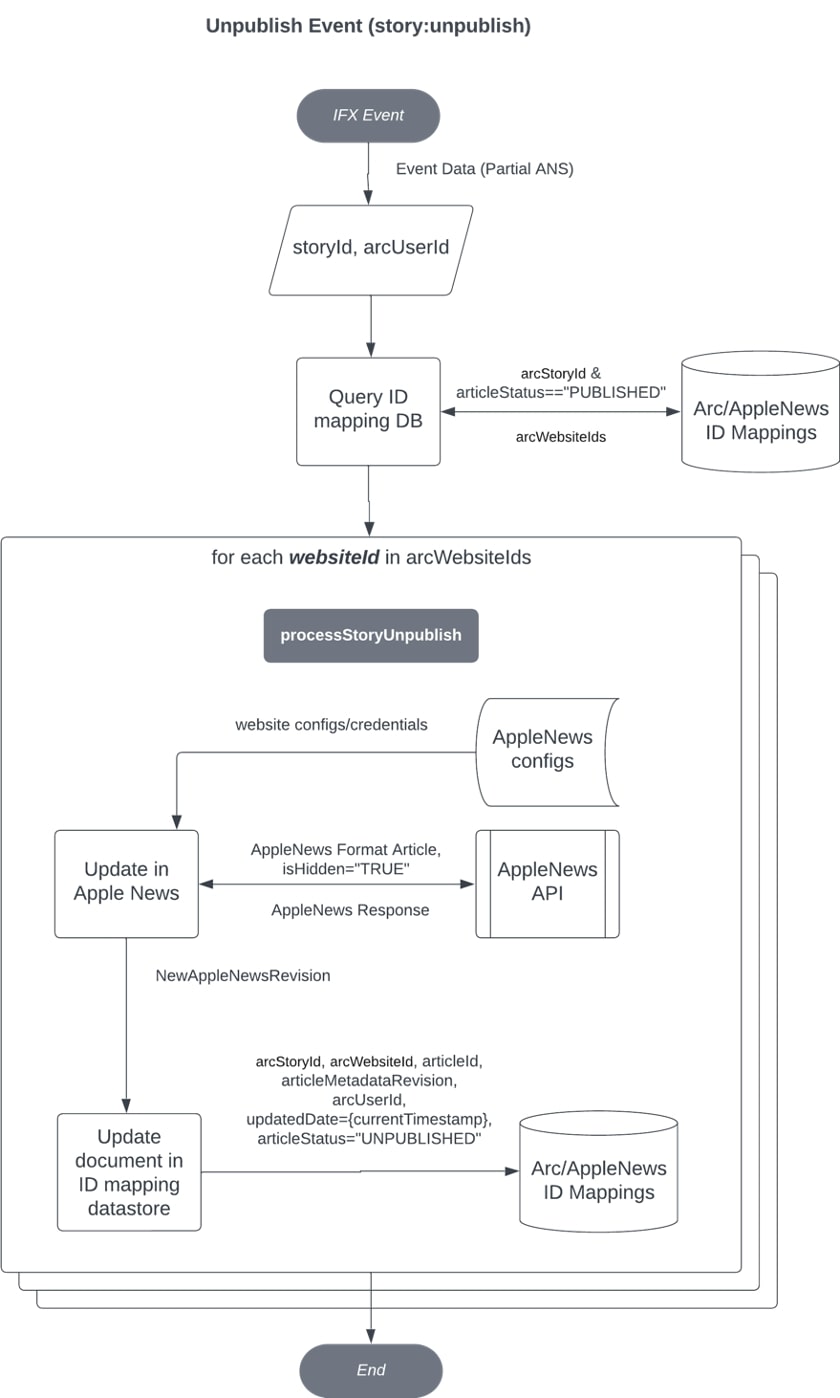
Delete Diagram:
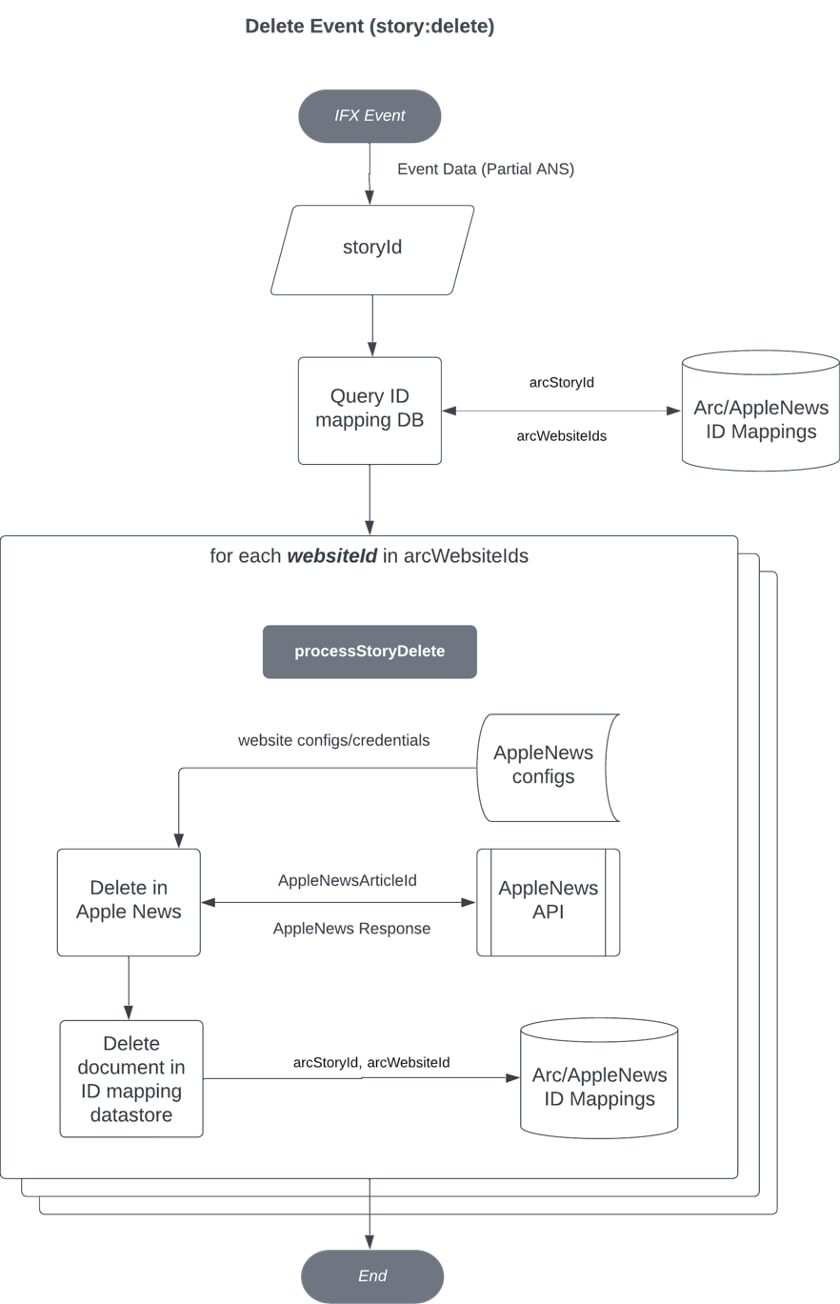
The proposed solution is robust enough to support multi-site organizations. Publishing (or republishing) a story that has been circulated to multiple websites pushes your content to each configured Apple News channel. Any articles with Arc XP websites or sections that are not configured are published to the default Main Apple News channel if configured.
It is also important to point out that we need to keep track of the relationship between Arc XP IDs, with their corresponding Apple News article IDs, along with other necessary data. Therefore, you must stand up a database and update this on specific publishing actions to adopt the proposed solution.
In later sections, we take a closer look at various key parts of the code, including the database requirements for the ID mapping.
Initial Setup
The following sections describe items that must be in place prior to using this solution.
Websites and Sections in Arc XP
Whether you are a long-standing customer on Arc XP or have just onboarded, at this stage you should have already set up websites and sections in Site Service. For more information on setting up websites and sections, see Managing Websites in Site Service.
iCloud News Publisher
Set up iCloud News Publisher. To publish content into Apple News, you must have the necessary credentials, as well as set up the necessary channel and sections.
Apple/iCloud Account
To log into iCloud, you need an Apple ID.
- Visit https://www.icloud.com/, and click Sign In.
- Sign in with your Apple ID. If you do not have an Apple ID, you can create one by clicking Create Apple ID and submitting the form.
As part of this process, you must enroll in the Apple Developer Program. You can achieve this by following Before You Enroll - Apple Developer Program. Ensure that your Apple ID and organization are created in a region that supports Apple News. For a comprehensive list, visit Availability of Apple Media Services.
Request the Necessary Channels
Contact your Apple representative requesting that they assist you with creating the channels that you need. Apple has advised us that each website (or brand) should be associated with its own Apple News channel. Keep in mind that if you want to test pushing stories to a Sandbox environment, you must request both channels for Sandbox websites as well as for Production websites. The naming convention of the channel is up to you, but we recommend including both the website and the environment in the name. The channel name could look something like: My Website - Sandbox or My Website - Production.
Ultimately, the list of channels should reflect the number of websites that your org has, both in Sandbox and Production. After you request the channels, Apple can send the invite to create the channels to only one Apple ID. As soon as the owner of this Apple ID creates all the channels, they can add other members and assign their role.
Note: If a particular Apple News channel needs to have content restricted behind a paywall, please communicate this to your Apple representative. As additional configuration will be required on the Apple side to enable this feature.
Channel Setup in Apple News
Now that you have the channels created and available in the iCloud News Publisher app, you can edit each channel so that it reflects the sections that have been created in Arc XP.
To edit each channel, see the Add and manage sections in News Publisher documentation.
IFX integration running locally
Follow the guide Create and Manage Integrations
Code & Database Setup
The following sections describe how to get started in your IFX to Apple News setup.
Starter Code
This document provides example code in a public GitHub repository. Developers created this code to serve as a starting point so that anyone can implement an Apple News integration in a short period of time. We cover the basic workflows, so for most customers, adopting this solution could be a simple matter of setting configurations and making minor adjustments to adhere to your particular workflow.
Fork this repository into your own repository. Do not use the package.json
from this code, use the package.json
file that was automatically generated when you created an integration.
Replace Placeholders
Search for the following placeholders throughout the codebase and replace them with the corresponding values:
<add_your_org_id>
- replace for your Arc XP org id as it appears in your arcpublishing.com URL (without environment prefix).<add_your_integration_name>
- replace for the name of your integration as set in the “Create the Integration” step.
Install Dependencies
In order to fully use the example code, you will have to install the following dependencies (or compatible versions): Dependencies
- axios 1.4.0
- cheerio 1.0.0-rc.12
- crypto-js 4.1.1
- dayjs 1.11.9,
- form-data 4.0.0
- pino 8.15.1
Install the following 2 only if you are using the DynamoDB solution for the ID mapping table provided in this example code:
- @aws-sdk/client-dynamodb 3.387.0
- @aws-sdk/lib-dynamodb 3.387.0
Dev Dependencies
- eslint 8.44.0
- eslint-config-standard 17.1.0
- eslint-plugin-import 2.27.5
- eslint-plugin-jest 27.2.2
- eslint-plugin-n 16.0.1
- eslint-plugin-promise 6.1.1
- husky 8.0.3
- jest 29.5.0
- npm-run-all 4.1.5
Copy npm Scripts
In the package.json
file from the example code, you will find some useful npm scripts that help with running, testing and bundling the integration. Copy these into your your own package.json file.
Set up a Database
As previously mentioned, to adopt this solution, we must persist data that ties the Arc XP story ID with its corresponding article ID in Apple News.
IFX invocations are fire-and-forget (stateless), so no data is persisted between separate invocations. For that reason, an ID Mapping table is required to keep track of what Arc ID corresponds to a particular Apple News article ID. You also need to track additional information, like the Apple News article revision.
You must track these ID mappings on a website-to-website basis. In other words, an Arc XP story ID that has been circulated to multiple websites is associated with multiple Apple News article IDs, a different ID for each website.
The starter code already includes an example database service that connects to a previously created DynamoDB table. For local development, we include commands that stand up a Docker container that runs a local instance of DynamoDB.
Nevertheless, you are not tied to this and can make use of whatever database service you prefer. We recommend that this table includes at least the following fields (field names appear here as they appear in the starter code):
FIELD | DESCRIPTION |
---|---|
arcStoryId | The Arc XP Universally Unique Identifier (Arc XP UUID) is the story ID. |
arcWebsiteId | The ID for the website that the arcStoryId was circulated to on publish. |
articleId | The Apple News article ID that was assigned to the story on publish. This ID is different for each website that the story was circulated to. |
articleMetadataRevision | This is a unique revision ID that the Apple News API returns on each operation. This revision ID is required in order to make a subsequent update to an article. |
articleStatus | This field indicates the status of an article. The possible values are:
|
arcUserId | The ID of the last user that performed the publish/unpublish action. Informative. |
createdDate | The date and time that the article was published for the first time. Informative. |
updatedDate | The date and time that the last publish action was performed. Informative. |
Here is a graphic representation of what this table may look like (you may have to scroll left/right):
arcStoryId | arcWebsiteId | articleId | articleMetadataRevision | articleStatus | arcUserId | createdDate | updatedDate |
---|---|---|---|---|---|---|---|
H3K3H28ABXLS88SHRT2ZOPPWN6 | the-daily-times | 6e64d48d-12a8-4d28-a5ad-7cf19371febf | AAAAAAAAAAD//////////w== | PUBLISHED | john.doe@dailytimes.com | 2023-08-15T21:38:00.566Z | 2023-08-15T22:38:00.566Z |
H3K3H28ABXLS88SHRT2ZOPPWN6 | business-insider | 9dd4d54d-89d4-7f6e-a7bd-66cd9370c81f | BBBBBBBBBBC//////////w== | PUBLISHED | jane.doe@buisinessinside.com | 2023-08-15T23:40:09.477Z | |
H3K3H28ABXLS88SHRT2ZOPPWN6 | the-daily-bugle | 39ca644a-001b-8ef5-09c9-aa2193ccb3b | CCCCCCCCCCA//////////w== | UNPUBLISHED | tim.tomas@thedailybugle.com | 2023-08-16T10:00:10.491Z | 2023-08-16T18:00:10.491Z |
Where:
- The story for the-daily-times has been republished (note there is an update time)
- The story for business-insider has been published only for the first time (note there is not an update time)
- The story for the-daily-bugle has been de-circulated (not a complete unpublish because the story is still published for the other websites)
The relevant code for these database operations is explained in more detail in a later section.
Integration Environment Variables
IFX projects include the .env.*
files for storing environment variable values and make these variable values available to the integration on deployment (or local runs). To successfully run the Apple News integration, you must set up some environment variables in these files.
Here is an example of one of these files:
# DO NOT STORE SECRETS IN THIS FILE. Secrets should be added via the Arc Admin API, not# checked in to source control.
INTEGRATION_NAME=my-apple-news-integrationNODE_ENV=sandboxLOG_LEVEL = INFO
ARC_CONTENT_API_URL=https://api.sandbox.example.arcpublishing.com/content/v4/ARC_PHOTO_API_URL=https://api.sandbox.example.arcpublishing.com/photo/api/v2/photos/RESIZER_PATH=/resizer/v2/CONTENT_API_REQUEST_DELAY=3000
THE_DAILY_TIMES_BASE_URL=https://dailytimes-staging-sandbox.web.arc-cdn.netBUSINESS_INSIDER_BASE_URL=https://businessinsider-staging-sandbox.web.arc-cdn.netTHE_DAILY_BUGLE_BASE_URL=https://dailybugle-staging-sandbox.web.arc-cdn.net
APPLE_NEWS_URL=https://news-api.apple.comAPPLE_NEWS_DEFAULT_ARTICLE_LANGUAGE=enAPPLE_NEWS_PUB_AWS_REGION=us-east-1
THE_DAILY_TIMES_APPLE_NEWS_CHANNEL_ID=102d1781-492f-4ab5-b18e-a2314a2f055fBUSINESS_INSIDER_APPLE_NEWS_CHANNEL_ID=ccc638ef-ac57-46a7-ba85-72d85e795c75THE_DAILY_BUGLE_APPLE_NEWS_CHANNEL_ID=80e3d509-9400-449c-9466-aea5a2469f2e
General Environment Variables
VARIABLE | DESCRIPTION |
---|---|
NODE_ENV | The node environment that this is running in the corresponding environment. The value for this variable can be production, sandbox, or development. |
INTEGRATION_NAME | The name of your integration as you created in the Create your integration section. |
RESIZER_PATH | A path to Resizer V2. This is a fixed value for all organizations. |
CONTENT_API_REQUEST_DELAY (optional) | When set, determines the number of milliseconds that the integration waits before making requests to Content API. If unset or the value is 0, no delay occurs. We recommend you set this variable to avoid possible race conditions between your integration and Content API updates. |
ARC_CONTENT_API_URL | The Content API base URL for your org. |
ARC_PHOTO_API_URL | The Content API base URL for your org. |
LOG_LEVEL | Determines the log level option used for Pino, a logging utility used in the project. You can find all the supported values in the Pino documentation. Additionally, for this integration we introduce the DISABLED value, which will silence all logging when set. |
APPLE_NEWS_URL | A fixed URL for Apple News. This does not change. |
APPLE_NEWS_DEFAULT_ARTICLE_LANGUAGE | The default language for your Apple News articles. |
APPLE_NEWS_PUB_AWS_REGION (optional) | If using the DynamoDB solution for the ID Mappings included in the starter code, you must set the AWS region that the table is located in. |
Website-specific Environment Variables
VARIABLE | DESCRIPTION |
---|---|
{WEBSITEID}_BASE_URL | Requires one entry per website. The entry should include the CDN URL for the website. The system uses this URL to construct a resizer URL for images. Using a resizer URL has some caching benefits that can boost performance when loading images. |
{WEBSITEID}_APPLE_NEWS_CHANNEL_ID | You need one entry per website. Each entry contains the website ID converted into all caps followed by a fixed _APPLE_NEWS_CHANNEL_ID . For example, if the website ID is the-daily-news , then the entry is THE_DAILY_NEWS_APPLE_NEWS_CHANNEL_ID . You can find the channel ID in the iCloud News Publisher. To read steps on how to retrieve this ID (alongside the Apple News API secrets that you set up in the following section), see Use your CMS with News Publisher. |
Secrets
The Apple News IFX integration requires the use of various keys for both the Arc XP APIs as well as the Apple News API. For Sandbox and Production, you must use the IFX Secrets Manager endpoint to set up the necessary secrets for all the APIs.
Conversely, for local development, you must set these keys as if they were environment variables in your .env
file. Remember that this file should NEVER be checked into source control.
Developer Access Tokens
Apple News makes use of the Content and Photo APIs. Therefore, in order to access these services, you must set up a secret read-only developer access token. To create a developer access token, see How to Use Developer Access Tokens to Access the Arc XP APIs.
After you create the developer access token, set up the developer access token as a secret (and in your .env
file for local development), naming the secret as ARC_TOKEN and assigning the value for the created token.
Apple News Secret Keys
You must also retrieve the Apple News Key ID and Key Secret for each one of the websites that you manage. To retrieve these values, access each channel in the iCloud News Publisher and view the credentials by following the steps described in Use your CMS with News Publisher.
After you have these keys, similar to the previous step, you must set these secrets by using the IFX secrets manager as well as add new entries into the .env
file for local development.
The name for these secrets should follow a similar structure for the channel IDs. For each website, you should have two entries with the following format:
{WEBSITEID}_APPLE_NEWS_KEY_ID
{WEBSITEID}_APPLE_NEWS_KEY_SECRET
And for each secret entry, add the value of the keys that you retrieved for that channel (website).
AWS Secret Keys (if applicable)
If you’re using the database implementation provided in the starter code, then you must set up secrets to store the credentials for the DynamoDB in AWS. For this, you will have to configure the following two fields as secrets:
{YOURINTEGRATIONNAME}_AWS_SECRET_ACCESS_KEY
{YOURINTEGRATIONNAME}_AWS_ACCESS_KEY_ID
Both fields include the access keys provided by AWS.
Lastly, name the table, taking into consideration the following items:
INTEGRATION_NAME + "-" + NODE_ENV + "-IdMappings"
where INTEGRATION_NAME
and NODE_ENV
match the values provided in the .env.*
files.
Apple News Configuration Files
To send articles to the Apple News API, the requests need to contain metadata. The values of these article metadata fields are determined by the developer through a configuration file. The value for these fields can be set at the organization, website, and section levels.
These article metadata are set through configuration files. Three different configuration files exist: one for local development, one for Sandbox, and lastly, one for Production.
The files are at the root level and can be identified as the files that have an apple-news-conf-{environment}.json
format, where environment can be development, sandbox, or production.
The starter code comes with an example data structure that you can edit and adapt to your configuration needs. The basic condensed structure of these JSON files looks something like this:
{ "orgMetadata": { "isCandidateToBeFeatured": false, "isHidden": false, "isSponsored": false, "isPreview": false, "isPaid": false, "maturityRating": "GENERAL", "targetTerritoryCountryCodes": [ "US" ] }, "websites": { "website-id-1" { "styling": { "componentLayouts": { // various class objects for component layouts objects }, "componentStyles": { // various class objects for component styles }, "componentTextStyles": { // various class objects for compoenent text styles }, "layout": { // layout options } }, "metadata": {}, "sections": { "default": { "name": "Main", "url": "https://news-api.apple.com/sections/bbf7ee17-96a0-4b67-add3-696c9a6cde43", "metadata": {} }, "/amazing-section": { "name": "Amazing Section", "url": "https://news-api.apple.com/sections/22330691-cab8-4c6a-94db-e3e2cbc0d9b7", "metadata": {} } } }, ... rest of website configurations }}
This is not a comprehensive example, but instead, it serves the purpose of displaying the key parts of this configuration file. The configuration file has a top level orgMetadata key, followed by a websites key. You must set configurations for each website. The key for each website must match the Arc XP website ID (as it appears in Site Service).
Now that we’ve seen the basic structure of this file, let’s set up the necessary configurations.
Metadata
The article metadata fields (not to be confused with the metadata object within the article document) is information that needs to be sent in the request payload. This information can determine several aspects of your story after it has been sent to Apple News. These aspects may have to do with visibility, sponsored content, target territories, and so on.
For a full description of the metadata fields, see Apple's Create Article Metadata Fields and the Update Article Metadata Fields documentation.
Not included in the documentation, there is an optional isPaid field that can be set to true to indicate that an article should be restricted behind a paywall. This metadata option is available only if Apple has configured this feature for your channel. The starter code has this set to false for the entire organization.
With the provided data structure, you can set the values at the organization level under the orgMetadata key. You can also set these values at the website level under the metadata key within each website. Lastly, you can set these values at the section level, under the metadata key within each section.
Note that section-level metadata overwrites both website- and organizational-level metadata, and website metadata takes precedence over the organization metadata.
Different sections within a website may have different metadata. Therefore, the primary section medatada is always the one selected to overwrite any higher-level metadata. If for some reason, no metadata configuration exists for the primary section, then the system considers the website- and organization-level metadata for the article metadata, ignoring any other section metadata.
Apple News Styling
With Apple News, you have total control over the appearance of your story when displayed in Apple News.
You must configure the styling at the website level under the styling key. Inside this key, you will find four style and layout options that ultimately determine the aspect of your article in Apple News:
KEY | DESCRIPTION |
---|---|
layout | This object defines columns, gutters, and margins for your article’s designed width. You can find a more detailed explanation on this object in Apple’s Layout documentation. |
componentLayouts | Contains a collection of objects that define the positioning for specific components within the article’s column system. You can find a complete description of the available options in Apple’s ComponentLayout documentation. |
componentStyles | Component styles are at the heart of creating a unique article with Apple News Format. They provide options that range from adding background images and drawing borders to setting the styles for the rows, columns, and cells in a table. You can find the full documentation on this option in Apple’s Component Styles documentation. |
componentTextStyles | Defining the style for a text component, including spacing, alignment, and drop caps. You can find more detailed information at Apple’s Component Text Style documentation. |
You can use classes that are defined in these styling configurations when building components. We describe this in more detail in the Understanding key parts of the code section.
Map Website Sections to Channel Sections
If you’ve completed the Channel setup in Apple News step in the Pre-requisites section, then you should have sections in Apple News that match your configured sections in Arc XP. Now we have to map the Arc XP sections to their corresponding section in Apple News.
If you observe the sections key in the previous JSON example, note that this is set at the website level, and also that it contains a list of sections. The individual section keys should match the section ID as it appears in Arc XP Site Service.
Within each section, you must set at least the following values:
KEY | DESCRIPTION |
---|---|
name | This is simply an illustrative name for the section. It can be any value. |
url | The URL for the section in Apple News. You can find this URL by clicking the link icon next to the section name in the iCloud News Publisher console and then copying the section ID in the popup and pasting it at the end of the https://news-api.apple.com/sections/ base URL. The result should look something like https://news-api.apple.com/sections/72836472-fcd0-5d7d-89af-b0f5aac9b1c1 . |
Optionally, you can also set metadata at the section level:
In the configuration and in Apple News, you also set a default section (which is main in the previous Apple News image). Having a configuration and Apple News section with this ID makes it so that, if for some reason, a section in Arc XP has not yet been added to the configuration file, it will link the story to this main section.
Running the Integration Locally
Running your Apple News integration is the same as running any other integration. If you need a refresher on how to do this, see Getting Integrations Running Locally.
The only prerequisite for running this particular integration is that you must ensure that the ID Mapping table (mentioned in the Prerequisites section) is accessible by your local application and that your database credentials are in place.
If you decided to use another database, you may also have to make changes to that particular service within the code.
We go into more detail into the ID mapping table in the Understanding key parts of the code section. We also share some example payloads for invoking the events locally. These examples contain the bare minimum that you must provide to your event handlers:
When invoking the First Publish handler:
{ "organizationId": "sandbox.yourOrg", "currentUserId": "john.doe@domain.com", "key": "story:first-publish", "typeId": 1, "body": { "_id": "5LSLD5VKBJBEZOSG4D5ZN6JDEQ", "_website_ids": ["website-id-1", "website-id-2"], ... rest of ANS (not including content elements) }, "version": 2, "uuid": ""}
When invoking the Republish handler:
{ "organizationId": "sandbox.yourOrg", "currentUserId": "john.doe@domain.com", "key": "story:story:republish", "typeId": 1, "body": { "_id": "5LSLD5VKBJBEZOSG4D5ZN6JDEQ", "_website_ids": ["website-id-1", "website-id-2"], ... rest of ANS (excluding content elements) }, "version": 2, "uuid": ""}
When invoking the Unpublish handler:
{ "organizationId": "sandbox.yourOrg", "currentUserId": "john.doe@domain.com", "key": "story:story:unpublish", "typeId": 1, "body": { "_id": "5LSLD5VKBJBEZOSG4D5ZN6JDEQ", ... rest of ANS (excluding content elements) }, "version": 2, "uuid": ""}
When invoking the Delete handler:
{ "organizationId": "sandbox.yourOrg", "currentUserId": "john.doe@domain.com", "key": "story:story:delete", "typeId": 1, "body": { "_id": "5LSLD5VKBJBEZOSG4D5ZN6JDEQ", ... rest of ANS (excluding content elements) }, "version": 2, "uuid": ""}
Note that in the cases of the unpublish and delete handlers, you don’t need the _website_ids
because we simply unpublish or delete in every website this story has been published to.
Subscribing to IFX events
Now that we are code and configuration complete, let’s subscribe to events in preparation for the deployment to live environments. You must subscribe your integration to the following events:
- story:first-publish
- story:update
- story:republish
- story:unpublish
- story:delete
If you need guidance on how to do this, see the IFX Events page.
Deploying the Integration
To deploy changes to Sandbox or Production, you must complete three steps:
- Bundle your code
- Deploy your bundle
- Promote your bundle to live (direct traffic to the newly deployed bundle)
The started code provided in this recipe has an npm command that helps with step 1.
Bundling your code is as simple as executing the npm run createSandboxBundle
or npm run createProdBundle
, depending on what environment you’re deploying to. This creates a zip file that you need in the next steps.
Steps 2-3 are described in greater detail in the Bundle deployment workflow document.
Unit Tests
The started code already provides test coverage and linting, though each adopter is free to add, edit, and remove tests as they see fit. Linting is also included. In order to run both the linting and the unit tests, you can use the npm run validate command.
You can also run linting and unit tests separately by executing npm run lint and npm run test, respectively.
Extending the Capabilities
The aforementioned guidelines and suggestions are true for the starter code. As maintainers of your own integration, you have total freedom of how your integration works and what you want it to do.
Understanding Key Parts of the Code
If adopters are going to be the maintainers of their integration, we consider it necessary walking you through key parts of the starter code Walking through the code gets adopting developers familiar enough so they can make their own adjustments and improvements to tailor to the needs of the business.
We provide you with an overview of the most notable services and utilities that are included in the starter code.
Services
Apple News API Service
In the starter code, we include a src/services/appleNewsAPI.js
service file.
This service is a wrapper for the Apple News API. This includes all the necessary code to perform the basic CRUD (create, read, update, and delete) operations in Apple News, such as: post an article, update an article, update the article metadata (used for hiding a story in Apple News on story unpublish) and delete articles.
You can find complete documentation in Apple's Apple News API documentation.
Content API and Photo API
Under that same folder (src/services), you also find two other files: contentApi.js
and photoAPI.js
.
These services include the code necessary to make requests to the Content API and Photo API, respectively.
The Content API can retrieve the full ANS of a story, given that by design the IFX event body does not contain the content_elements property. The content_elements property is required in order to transform an Arc XP story into its Apple News Format equivalent.
The Photo API is called for a particular use case, where a user may insert an HTML content element that contains an image as a hyperlink. Because the src attribute for this image only includes a Cloudfront URL, we have to parse out the ID from the URL and then query the Photo API for the full image ANS. This is not a common use case, but also not impossible.
If the business needs require reaching out to other Arc XP services, adopters can follow a similar pattern as the one used for these services.
ID Mapping Repository
As mentioned in the Set up a database section, an ID mapping database is required in order to keep a relationship of a given Arc XP story ID with its corresponding Apple News article ID for each website.
The ID Mapping Repository Service, located in src/services/idMappingRepository.js
, encompasses all the logic needed to perform the basic CRUD operations to this database.
In the starter code, we provide an example using AWS’s DynamoDB, though if you want to use another database service, you can change this. But we recommend ensuring that the function names remain the same so that the change is transparent throughout the rest of the code; however, you aren’t bound by this change and can change it at any time.
Utilities
Transformation from ANS to Apple News Format
When sending a story to Apple News, you need to transform the ANS into Apple News Format.
In the src/utils/appleNewsHelpers.js
file, you can find numerous utility functions that enable our integration to support this transformation.
Arc XP wrote this module taking into consideration the most common use cases and recommendations from the Apple team. Though if you want to alter the way that the transformation is being made, you can make the change here.
The main function that is called to perform the transformation is ansToAppleNews
. This function receives the full ANS of the story and returns an Apple News article document.
Within this same file, you can find that this output is segmented through the use of various functions.
The buildAppleNewsBaseArticle
function converts the root level fields of the Apple News article document.
The function that performs most of the transformation is the buildComponents
function, which in turn calls the buildHeaderComponents
and buildContentElementComponents
functions. These functions are in charge of creating the components for your Apple News article. Think of components as the building blocks for your content in Apple News. You can read more about Apple News Components in Apple’s Components documentation.
The buildHeaderComponents
function creates a first compound component that is made up of other components. For the starter code, it is made up by the title of the story, followed by a particular image (a promo item image, or if it is not set, then the first content element if it’s an image) if available, with its respective photo author and/or caption. Lastly, the header ends with two bylines including the author of the article and the publish date.
The buildContentElementComponents
goes through all the content elements in the story and converts them into its corresponding Apple News Format equivalent. Depending on the type of content element, it calls a specific build{****}Component
function, which receives the ANS of the content element and returns an Apple News component.
Note that the starter code contains only a build{****}Component
function for the most commonly-used content elements.
The Apple News article object also contains a metadata property. This property is set by the buildMetadata function (NOT to be confused with the Apple News Metadata fields mentioned in the Set up Apple News configuration files -> Set up Metadata section).
In the Apple News article object, you also see the layouts, componentLayouts
, componentStyles
, and componentTextStyles
properties, which are all populated by the styles that were set in the Set up Apple News configuration files -> Apple News Styling section.
Lastly, this file contains a buildAppleNewsArticleMetadata
function that assists with constructing the Apple News article fields object that is sent in the request. The data for this object is determined by whatever you set in Set up Apple News configuration files -> Set up Metadata.
ANS Helpers
In the src/utils/ansHelpers.js
file, you find various utility functions that help with the manipulation of ANS.
Troubleshooting
This section provides some of the most commonly-identified scenarios that could produce unexpected behavior, along with possible causes and mitigation steps.
SCENARIO | POSSIBLE CAUSE | MITIGATION STEPS |
---|---|---|
My story is not showing up in Apple News | Your integrations are not subscribed to the necessary events | Ensure that you are subscribed to the events indicated in the Subscribe to Events section. If this was the root cause and you have subscribed to all the events, republish the story. |
A runtime error exists in your deployed code | Consult the IFX logs to see if there is an error occurring on runtime that is preventing your story from being published. If an error appears, correct the error, re-deploy the integration, and update the publish of the story. | |
A Content API rate limit has been reached | Consult the logs to see if this is the case. If this was the culprit, wait for a few minutes and republish the story. If a story received edits between the time the story was published and the time the issue was identified, then additional steps may be required:
| |
My story is not updating (or publishing) in Apple News, despite already being published and appearing in Apple news | Your integrations are not subscribed to the necessary events | Ensure that you are subscribed to the events indicated in the Subscribe to Events section. If this was the root cause and you have subscribed to all the events, republish the story. |
A runtime error exists in your deployed code | Consult the IFX logs to see if there is an error occurring on runtime that is preventing your story from being published. If an error appears, then correct the error, re-deploy the integration, and update the publish of the story. | |
Your story was not saved in the ID mappings table | Check the ID mappings table to make sure that the ID mapping for that story ID and website was saved correctly. If the record is missing, it’s possible that the database operation failed when publishing the story for the first time. If that is the case, make sure that the database is available. Then simply delete the Apple News article and update the publish in Arc XP. Updating the publish creates a new article and inserts the corresponding record in the database. All subsequent updates should work as normal. | |
An Apple News article did not delete automatically when the story was deleted in Arc XP | Your integrations are not subscribed to the necessary events | Ensure that you are subscribed to the events indicated in the Subscribe to Events section. If this was the root cause and you have subscribed to all the events, republish the story. |
A runtime error exists in your deployed code | Consult the IFX logs to see if an error is occurring on runtime that is preventing your story from being published. If an error appears, then correct the error, re-deploy the integration, and update the publish of the story. | |
Your story was not saved in the ID mappings table | The record for the ID mapping may have never been inserted. If a story was not deleted automatically, you can simply go to the iCloud News Publisher console and delete the article manually by following the steps in Apple’s Delete an article documentation. | |
My article does not reflect any changes, despite being subscribed to the events, has no errors in the logs, and the ID mappings table has the record for this story ID and website saved correctly | Content API is not synched with the latest publish revision | Without making edits, update the publish of your story. This should reflect the latest published content in Apple News. |